By default WcfStorm does a field-by-field comparison of the actual and expected response of a functional test case. This behavior is appropriate for a large number of scenarios. However, in some cases however, you might want to have control the validation process. For example, let's say you have a WCF service that returns a GUID parameter which is generated everytime the method is invoked. If you've created a test case for this method, then the test case would always "fail" because the GUID in the expected response will never match the one coming from the actual response. Having full control on the validation process makes sense for such a scenario.
Controlling the validation process in WCFStorm is accomplished by creating a validation plugin that implements IFunctionalTestValidator. Below is the signature of IFuntionalTestValidator
public delegate void PluginLoggingHandler(object sender, string logMsg, PluginLogType logtype);
public enum PluginLogType
{
Error,
Success,
Info,
Warning,
Debug
}
public interface IFunctionalTestValidator
{
bool OverrideDefaultValidation{get;}
event PluginLoggingHandler LogMsg;
}
- PluginLoggingHandler LogMsg :
This event is used for printing log messages to the log window of WCFStorm. There are 5 types of log messages (as shown in the enum PluginLogType). Each log type corresponds to a different color, Error = red, Success = blue, Info =black etc.)
- bool OverrideDefaultValidation :
This property is used to indicate that the plugin will override (set to True) or supplement (set to False) the validation.
When the default validation is overriden, field-by-field matching will not be done and the status of the test case depends entirely on the custom validation(s). If set to false, the default validation will be executed together with all custom validations
Steps to create a custom validation plugin
Let's say we have the simple WCF service below and we'd like to write custom validations for the method "GetDataUsingDataContract". We'd like to validate that comp.StringValue is a concatenation of the stringValue and booleanValue input paramaters. For this, we'll create a custom validation.
public class Service1 : IService1
{
public int GetData(int value)
{
return value;
}
public CompositeType GetDataUsingDataContract(bool boolValue, string stringValue)
{
var com = new CompositeType();
com.BoolValue = boolValue;
com.StringValue = String.Format("{0}-{1}",stringValue, boolValue);
return com;
}
}
To create the validation plugin follow the following steps. (code download at the bottom)
- Create a functional test cases named TestGetDataUsingDataContract (can be any name)
- Create a C# library project. Reference WcfStorm.Plugins.dll (located in the same folder as wcfstorm.exe) and set Copy Local to True.
- Implement IFunctionalTestValidator.
- Add custom validation methods.
Custom validation methods return a boolean (true, means validation is successful) and is decorated with the TestCaseValidator attribute. This atrribute requires the MethodName and TestCaseName. The TestCaseName value should match the value of the test case created in step #1.
[TestCaseValidator(
MethodName = "GetDataUsingDataContract",
TestCaseName = "TestGetDataUsingDataContract",
Description = "BoolValue should be true")]
public bool ValidateGetDataUsingDataContract_FieldsMatch(string inputXml, string actualXml, string expectedXml, double elapsed)
The screenshot below shows the relationship between the test case and the custom validation method. (click to enlarge)
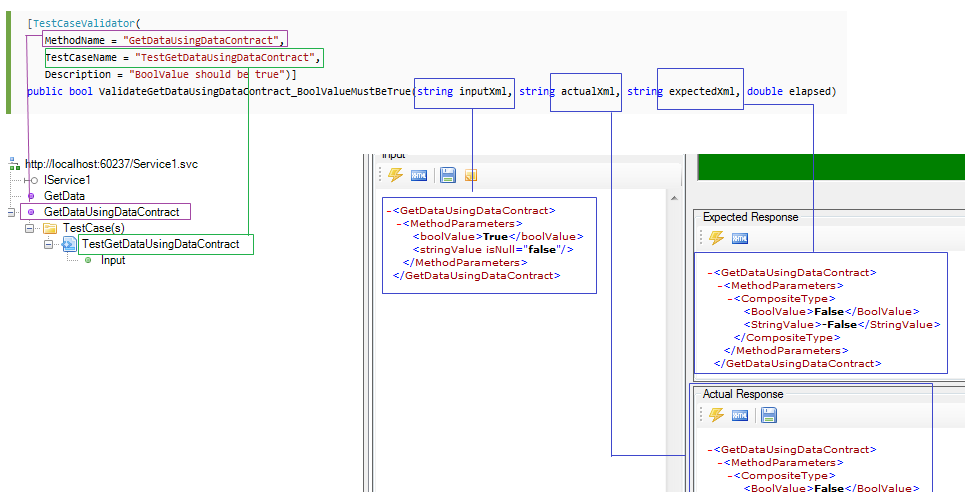
Below is the implementation of the "ValidateGetDataUsingDataContract_FieldsMatch" function which checks that the comp.StringValue is the concatenation of the boolean and string input parameters.
[TestCaseValidator(
MethodName = "GetDataUsingDataContract",
TestCaseName = "TestGetDataUsingDataContract",
Description = "String should be {str}-{bool}")]
public bool ValidateGetDataUsingDataContract_FieldsMatch(string inputXml, string actualXml, string expectedXml, double elapsed)
{
OnLogMsg("Starting custom validation: ValidateGetDataUsingDataContract_FieldsMatch", PluginLogType.Info);
var actualDoc = new XmlDocument();
actualDoc.LoadXml(actualXml);
var actualXpStringValue = "/GetDataUsingDataContract/MethodParameters/CompositeType/StringValue";
var actualValue = actualDoc.SelectSingleNode(actualXpStringValue).InnerText;
var inputDoc = new XmlDocument();
inputDoc.LoadXml(inputXml);
var inputXpBoolValue = "/GetDataUsingDataContract/MethodParameters/boolValue"; //Note the lower case "b" in boolValue
var inputXpStringValue = "/GetDataUsingDataContract/MethodParameters/stringValue";
var inputBoolValue = inputDoc.SelectSingleNode(inputXpBoolValue).InnerText;
var inputStringValue = inputDoc.SelectSingleNode(inputXpStringValue).InnerText;
var expectedReturn = String.Format("{0}-{1}", inputStringValue, inputBoolValue);
OnLogMsg(String.Format("Comparing expected vs actual : \"{0}\" == \"{1}\"", expectedReturn, actualValue), PluginLogType.Info);
return expectedReturn == actualValue;
}
- Compile the project.
- Load the dll into WCFStorm. If all goes well, you should see step#4. (click to enlarge)
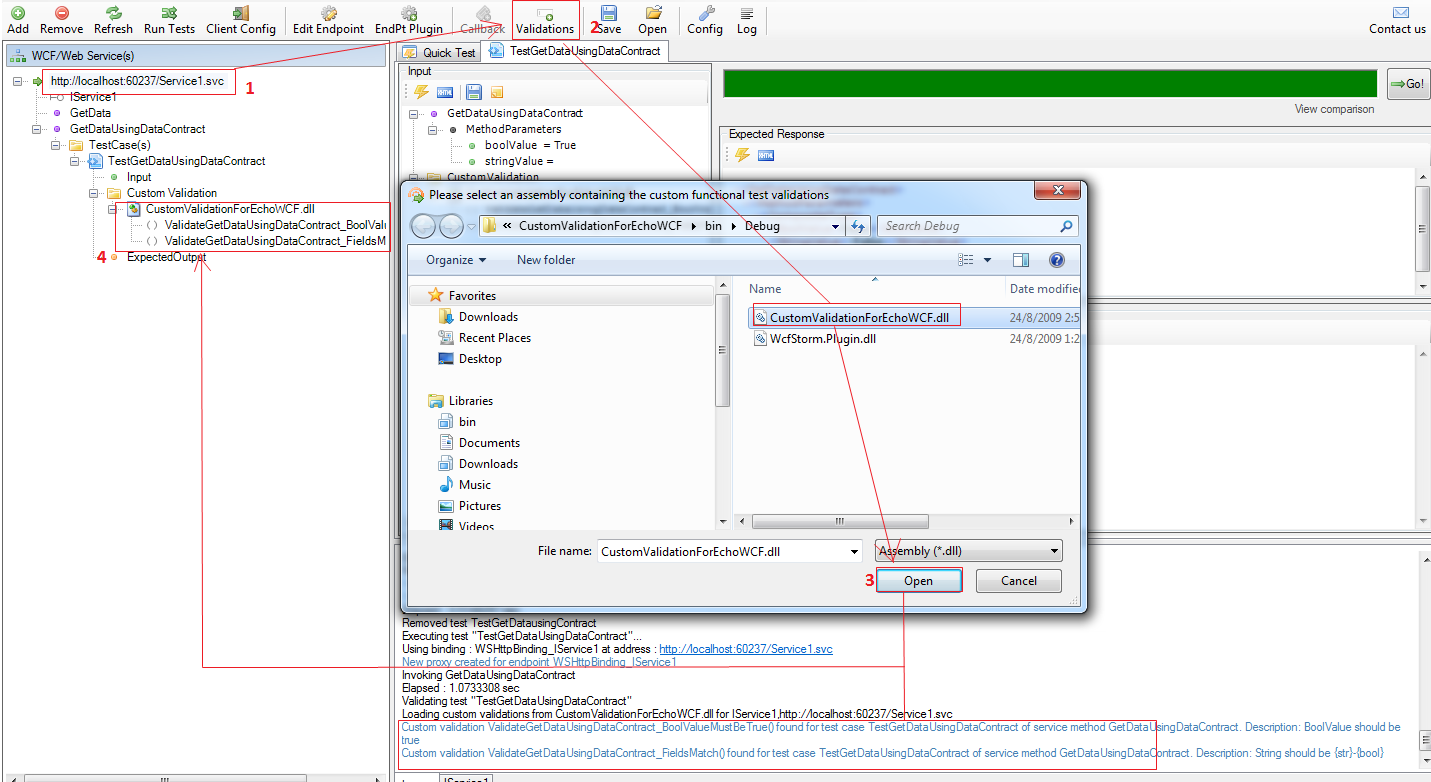
- Execute the functional test case.
Download sample code.